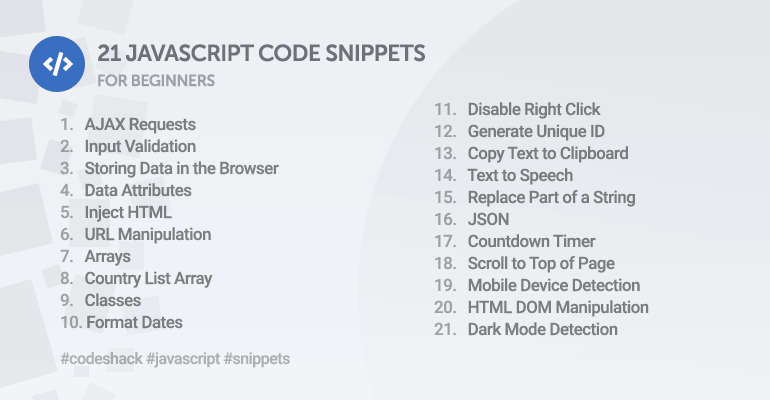
A comprehensive collection of code snippets you can incorporate into your projects. Ideal for beginners wanting to enhance their JS knowledge.
What is JavaScript?
JavaScript is a client-side scripting language used to develop interactive web applications. With JavaScript, you can dynamically update content on a webpage, animate elements, control media, and manipulate behaviour.
Contents
- AJAX Request
- Input Validation
- Storing Data in the Browser
- Data Attributes
- Inject HTML
- URL Manipulation
- Arrays
- Country List Array
- Classes
- Format Dates
- Disable Right Click
- Generate Unique ID
- Copy Text to Clipboard
- Text to Speech
- Replace Part of a String
- JSON
- Countdown Timer
- Scroll to Top of Page
- Mobile Device Detection
- HTML DOM Manipulation
- Dark Mode Detection
1. AJAX Requests
The following snippets will leverage the fetch() function to execute HTTP requests (GET, POST, etc.).
GET Request
Get a JSON file and output the contents:
fetch('https://example.com/data.json').then(response => response.json()).then(data => console.log(data));
POST Request
Execute a POST request with JSON declared as the content type:
fetch('https://example.com/authenticate', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ username: 'David', password: '12345' })
}).then(response => response.json()).then(data => {
console.log(data);
}).catch(error => {
console.error('Error:', error);
});
Using the FormData interface:
const formData = new FormData();
formData.append('username', 'David');
formData.append('password', '12345');
fetch('https://example.com/authenticate', {
method: 'POST',
body: formData
}).then(response => response.json()).then(data => {
console.log(data);
}).catch(error => {
console.error('Error:', error);
});
In addition to above, you can select an existing HTML form with:
const formData = new FormData(document.querySelector('#yourFormId'));
2. Input Validation
The following snippets will validate various input values.
Validate Email Address
if (/\S+@\S+\.\S+/.test('david@codeshack.io')) {
console.log('Email is valid!');
} else {
console.log('Email is invalid!');
}
String Length
Validate string length between two numbers:
const username = "david123";
if (username.length > 4 && username.length < 16) {
console.log('Username is valid!');
} else {
console.log('Username must be between 5 and 15 characters long!');
}
Alphanumeric Validation
Check if a string contains only letters and numerals:
if (/^[a-zA-Z0-9]+$/.test(username)) {
console.log('Username is valid!');
} else {
console.log('Username must contain only letters and numerals!');
}
Variable is Number
Check if a variable is a number:
const num = 12345.12;
if (!isNaN(parseFloat(num)) && isFinite(num)) {
console.log('Variable is a number!');
} else {
console.log('Variable is not a number!');
}
3. Storing Data in the Browser
The Web Storage API will enable us to preserve data in the browser. The localStorage interface can store up to 5MB per domain (in most browsers) as opposed to the 4KB limit cookies can store.
Set Item
Store a new item in local storage:
localStorage.setItem('username', 'David');
Get Item
Retrieve an item from local storage:
console.log(localStorage.getItem('username'));
Item Exists
Check if item exists in local storage:
if (localStorage.getItem('username')) {
console.log('Item exists!');
}
Remove Item
Remove an item from local storage:
localStorage.removeItem('username');
Remove all items:
localStorage.clear();
4. Data Attributes
The data attributes will enable you to associate data to a particular element. You can then subsequently use JS to retrieve the data.
The following snippet will retrieve data attributes with JS:
<a href="#" data-name="David" data-surname="Adams" data-unique-id="30" data-btn>Click me!</a>
const btn = document.querySelector('a[data-btn]');
btn.onclick = event => {
event.preventDefault();
// Output data attributes
console.log(btn.dataset.name); // David
console.log(btn.dataset.surname); // Adams
console.log(btn.dataset.uniqueId); // 30
};
5. Inject HTML
The following snippets will inject HTML into a specific point in the HTML document.
Inject After
Inject HTML after the given element:
<a id="home-btn" href="home.html">Home</a>
document.querySelector('#home-btn').insertAdjacentHTML('afterend', '<a href="about.html">About</a>');
Inject Before
Inject HTML before the given element:
<section id="section-2">
<h2>Title 2</h2>
<p>Description 2</p>
</section>
document.querySelector('#section-2').insertAdjacentHTML('beforebegin', `
<section>
<h2>Title 1</h2>
<p>Description 1</p>
</section>
`);
Append and Prepend HTML
Append an item to a list element:
<ul id="list">
<li>Item 2</li>
<li>Item 3</li>
<li>Item 4</li>
</ul>
document.querySelector('#list').insertAdjacentHTML('beforeend', '<li>Item 5</li>');
Prepend an item to a list element:
document.querySelector('#list').insertAdjacentHTML('afterbegin', '<li>Item 1</li>');
6. URL Manipulation
The following snippets will show you how to manipulate the URL.
Get Params
Get parameters from a URL:
const url = new URL('https://example.com/index.php?name=David&surname=Adams');
console.log(url.searchParams.get('name')); // David
console.log(url.searchParams.get('surname')); // Adams
Redirect URL
location.href = "https://codeshack.io";
Redirect in 10 seconds:
setTimeout(() => location.href = "https://codeshack.io", 10000);
Get Hash Value
// URL: http://example.com/home#MyProfile
console.log(window.location.hash.substr(1)); // Output: MyProfile
7. Arrays
In a nutshell, JavaScript arrays are an ordered list of values with each element specified as an index. You can store mixed values in an array, such as a string, integer, boolean, objects, and most other types.
Add Item to Array
The following snippet will append an item to an array:
const fruits = ['Apple', 'Orange', 'Pineapple'];
// Add to the end of the array
fruits.push('Strawberry');
Append multiple items:
fruits.push('Pear', 'Blueberry');
Prepend items to an array:
fruits.unshift('Blueberry', 'Kiwi');
Remove Item From Array
Remove the first item from an array:
fruits.shift();
Remove the last item from an array:
fruits.pop();
Array Validation
Check if an array contains an item:
if (fruits.includes('Pear')) {
console.log('Fruit array contains Pear');
}
Loop Array
Iterate an array and output the result:
fruits.forEach(item => console.log(item));
Get Random Item from Array
let fruit = fruits[Math.floor(Math.random()*fruits.length)];
Manipulate Array with Map
const newFruits = fruits.map(item => item + ' is a fruit.');
Reverse Array
const reversedFruits = fruits.reverse();
8. Country List Array
A populated array containing all the countries in the world:
const countries = ["Afghanistan", "Albania", "Algeria", "American Samoa", "Andorra", "Angola", "Anguilla", "Antarctica", "Antigua and Barbuda", "Argentina", "Armenia", "Aruba", "Australia", "Austria", "Azerbaijan", "Bahamas", "Bahrain", "Bangladesh", "Barbados", "Belarus", "Belgium", "Belize", "Benin", "Bermuda", "Bhutan", "Bolivia", "Bosnia and Herzegowina", "Botswana", "Bouvet Island", "Brazil", "British Indian Ocean Territory", "Brunei Darussalam", "Bulgaria", "Burkina Faso", "Burundi", "Cambodia", "Cameroon", "Canada", "Cape Verde", "Cayman Islands", "Central African Republic", "Chad", "Chile", "China", "Christmas Island", "Cocos (Keeling) Islands", "Colombia", "Comoros", "Congo", "Congo, the Democratic Republic of the", "Cook Islands", "Costa Rica", "Cote d'Ivoire", "Croatia (Hrvatska)", "Cuba", "Cyprus", "Czech Republic", "Denmark", "Djibouti", "Dominica", "Dominican Republic", "East Timor", "Ecuador", "Egypt", "El Salvador", "Equatorial Guinea", "Eritrea", "Estonia", "Ethiopia", "Falkland Islands (Malvinas)", "Faroe Islands", "Fiji", "Finland", "France", "France Metropolitan", "French Guiana", "French Polynesia", "French Southern Territories", "Gabon", "Gambia", "Georgia", "Germany", "Ghana", "Gibraltar", "Greece", "Greenland", "Grenada", "Guadeloupe", "Guam", "Guatemala", "Guinea", "Guinea-Bissau", "Guyana", "Haiti", "Heard and Mc Donald Islands", "Holy See (Vatican City State)", "Honduras", "Hong Kong", "Hungary", "Iceland", "India", "Indonesia", "Iran (Islamic Republic of)", "Iraq", "Ireland", "Israel", "Italy", "Jamaica", "Japan", "Jordan", "Kazakhstan", "Kenya", "Kiribati", "Korea, Democratic People's Republic of", "Korea, Republic of", "Kuwait", "Kyrgyzstan", "Lao, People's Democratic Republic", "Latvia", "Lebanon", "Lesotho", "Liberia", "Libyan Arab Jamahiriya", "Liechtenstein", "Lithuania", "Luxembourg", "Macau", "Macedonia, The Former Yugoslav Republic of", "Madagascar", "Malawi", "Malaysia", "Maldives", "Mali", "Malta", "Marshall Islands", "Martinique", "Mauritania", "Mauritius", "Mayotte", "Mexico", "Micronesia, Federated States of", "Moldova, Republic of", "Monaco", "Mongolia", "Montserrat", "Morocco", "Mozambique", "Myanmar", "Namibia", "Nauru", "Nepal", "Netherlands", "Netherlands Antilles", "New Caledonia", "New Zealand", "Nicaragua", "Niger", "Nigeria", "Niue", "Norfolk Island", "Northern Mariana Islands", "Norway", "Oman", "Pakistan", "Palau", "Panama", "Papua New Guinea", "Paraguay", "Peru", "Philippines", "Pitcairn", "Poland", "Portugal", "Puerto Rico", "Qatar", "Reunion", "Romania", "Russian Federation", "Rwanda", "Saint Kitts and Nevis", "Saint Lucia", "Saint Vincent and the Grenadines", "Samoa", "San Marino", "Sao Tome and Principe", "Saudi Arabia", "Senegal", "Seychelles", "Sierra Leone", "Singapore", "Slovakia (Slovak Republic)", "Slovenia", "Solomon Islands", "Somalia", "South Africa", "South Georgia and the South Sandwich Islands", "Spain", "Sri Lanka", "St. Helena", "St. Pierre and Miquelon", "Sudan", "Suriname", "Svalbard and Jan Mayen Islands", "Swaziland", "Sweden", "Switzerland", "Syrian Arab Republic", "Taiwan, Province of China", "Tajikistan", "Tanzania, United Republic of", "Thailand", "Togo", "Tokelau", "Tonga", "Trinidad and Tobago", "Tunisia", "Turkey", "Turkmenistan", "Turks and Caicos Islands", "Tuvalu", "Uganda", "Ukraine", "United Arab Emirates", "United Kingdom", "United States", "United States Minor Outlying Islands", "Uruguay", "Uzbekistan", "Vanuatu", "Venezuela", "Vietnam", "Virgin Islands (British)", "Virgin Islands (U.S.)", "Wallis and Futuna Islands", "Western Sahara", "Yemen", "Yugoslavia", "Zambia", "Zimbabwe"];
Populate a HTML form list:
<select id="countries"></select>
countries.forEach(country => document.querySelector('#countries').add(new Option(country, country)));
9. Classes
JavaScript classes are templates that consist of methods, variables, and inheritance.
Create Class
The following snippet will create a new class that will consist of methods and variables.
class Fruit {
constructor(name, color) {
this._name = name;
this._color = color;
}
eat() {
console.log('You ate the ' + this.name + '.');
}
get name() {
return this._name;
}
set name(name) {
this._name = name;
}
get color() {
return this._color;
}
set color(color) {
this._color = color;
}
}
Create a new instance:
let apple = new Fruit('Apple', 'Green');
Execute a method:
apple.eat(); // Output: You ate the Apple.
Get property:
console.log(apple.color); // Output: Green
Set property:
apple.color = 'red';
console.log(apple.color); // Output: red
Create a child class:
class Orange extends Fruit {
constructor() {
super('Orange', 'Orange');
}
throw() {
console.log('You threw the ' + this.name + '.');
}
}
Create a new instance of the child class:
let orange = new Orange();
orange.throw(); // Output: You threw the Orange.
console.log(orange.color); // Output: Orange
10. Format Dates
The following snippet will format a date in DD-MM-YYYY HH:MM format:
const date = new Date();
const dateString = date.getDate() + '-' + (date.getMonth()+1) + '-' + date.getFullYear() + ' ' + date.getHours() + ':' + date.getMinutes();
console.log(dateString); // Output: 26-1-2022 16:50
Assign the current date to an input date element:
<input type="date" id="input-date">
document.querySelector('#input-date').value = new Date().toISOString().substring(0, 10);
Assign the current date and time to an input datetime-local element:
<input type="datetime-local" id="input-datetime">
document.querySelector('#input-datetime').value = (new Date(date.getTime() - date.getTimezoneOffset() * 60000).toISOString()).slice(0, -1).split('.')[0];
11. Disable Right Click
The following snippet will disable right click:
document.addEventListener('contextmenu', event => event.preventDefault());
12. Generate Unique ID
The following snippet will generate a unique identifier:
const uniqueId = Date.now().toString(36) + Math.random().toString(36).substr(2);
console.log(uniqueId);
13. Copy Text to Clipboard
The following snippet will copy text to the clipboard:
<input type="text" id="input-text" value="You have copied this text!">
document.querySelector('#input-text').select();
document.execCommand('copy');
New API (may not be supported in all browsers):
navigator.clipboard.writeText(document.querySelector('#input-text').value);
14. Text to Speech
The following snippet will leverage the speech API and convert text to speech:
const speech = new SpeechSynthesisUtterance();
speech.lang = 'en-US';
speech.text = 'Hello World!';
speech.volume = 1;
speech.rate = 1;
speech.pitch = 1;
window.speechSynthesis.speak(speech);
15. Replace Part of a String
The following snippet will replace a substring with a new string:
const string = 'Hello, David!';
const replacedString = string.replace('David', 'John');
console.log(replacedString) // Output: Hello, John!
And to check if a string contains a substring, you can do:
if (string.includes('David')) {
console.log('String contains David');
}
16. JSON
JSON consists of text that represents arrays, objects, and other types of data.
The following snippet will convert an array to JSON text:
let contacts = [
{
'name': 'David',
'surname': 'Adams'
},
{
'name': 'John',
'surname': 'Doe'
}
];
console.log(JSON.stringify(contacts));
Convert JSON text to a JS object:
console.log(JSON.parse('[{"name": "David","surname": "Adams"},{"name": "John","surname": "Doe"}]'));
17. Countdown Timer
The following snippet will countdown a number in an element:
<p id="num">100</p>
const num = document.querySelector('#num');
setInterval(() => num.innerHTML = parseInt(num.innerHTML) != 0 ? parseInt(num.innerHTML)-1 : 0, 1000);
18. Scroll to Top of Page
The following snippet will scroll to the top of the page:
window.scroll({
top: 0,
left: 0,
behavior: 'smooth'
});
Old method:
document.body.scrollTop = document.documentElement.scrollTop = 0;
19. Mobile Device Detection
The following snippet will detect if the visitor is using a mobile device:
const isMobile = /iPhone|iPad|iPod|Android|Opera Mini|BlackBerry|IEMobile|WPDesktop|Windows Phone|webOS|/i.test(navigator.userAgent);
if (isMobile) {
console.log('Device is mobile');
}
20. HTML DOM Manipulation
The following snippets will show you how to manipulate HTML DOM elements.
Check if an element is visible:
const element = document.querySelector('#element');
if (element.offsetParent === null) {
console.log('Element is hidden');
}
Focus an element:
element.focus();
Remove focus:
element.blur();
Remove element:
element.remove();
Add class to an element:
element.classList.add('foo');
Remove class:
element.classList.remove('foo');
Toggle class:
element.classList.toggle('foo');
Get the selected item in a list:
<select id="list">
<option value="1">Apple</option>
<option value="2" selected>Orange</option>
<option value="3">Pineapple</option>
</select>
const list = document.querySelector('#list');
console.log(list.value); // Output: 2
console.log(list.options[list.selectedIndex].text); // Output: Orange
Check checkbox state:
<input type="checkbox" id="checkbox">
if (document.querySelector('#checkbox').checked) {
console.log('Checkbox is checked');
}
21. Dark Mode Detection
The following snippet will detect if the client has their theme preference set to dark mode:
const isDarkMode = window.matchMedia && window.matchMedia('(prefers-color-scheme: dark)').matches;
if (isDarkMode) {
console.log('Dark mode is enabled');
}
And to detect light mode:
const isLightMode = window.matchMedia && window.matchMedia('(prefers-color-scheme: light)').matches;
if (isLightMode) {
console.log('Light mode is enabled');
}
Conclusion
That's all the snippets I can think of from the top of my head. If you have any snippets you would like to share, drop a comment below.
If you have enjoyed reading this article, consider sharing it on social media and help us spread the word as it will help us create more quality content.
Enjoy coding!