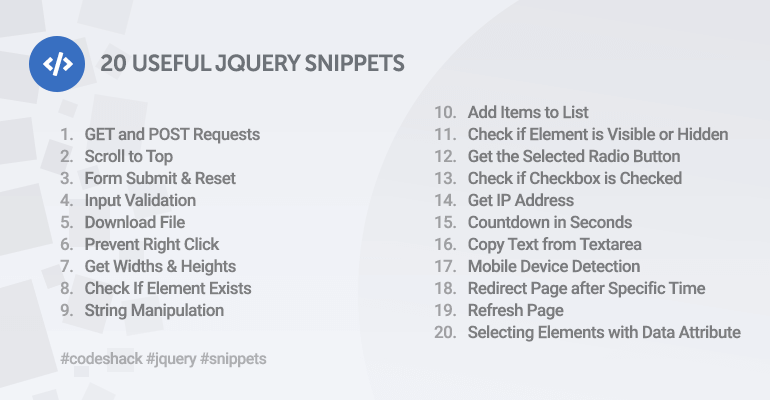
A common list of jQuery snippets you can seamlessly implement into your projects with minimal effort, whether your project is small or large, our snippets are convenient for all projects.
The snippets outlined in this article require the jQuery library. jQuery is a JavaScript library that makes the development of JavaScript applications easier, and it's the most popular JavaScript library used today, with over 78% of websites using it. I've personally been using it for projects for over ten years, almost near the initial release date back in 2006.
Contents
- GET and POST Requests
- Scroll to Top
- Form Submit & Reset
- Input Validation
- Download File
- Prevent Right Click
- Get Widths & Heights
- Check If Element Exists
- String Manipulation
- Add Items to List
- Check if Element is Visible or Hidden
- Get the Selected Radio Button
- Check if Checkbox is Checked
- Get IP Address
- Countdown in Seconds
- Copy Text from Textarea
- Mobile Device Detection
- Redirect Page after Specific Time
- Refresh Page
- Selecting Elements with Data Attribute
1. GET and POST Requests
The following snippet will execute a GET and POST request to a server using AJAX. You can easily make requests to your PHP applications or any other server-side programming language with this snippet.
$.get("example.php?name=david", function(data, status) {
// output the result to console
console.log(data, status);
});
$.post("example.php", {
"name": "David"
}, function(data, status) {
// output the result to console
console.log(data, status);
});
2. Scroll to Top
The following snippet will scroll to the top of a page with a click of a button, with added animation.
$(".scrolltotop").click(function(event) {
event.preventDefault();
$("html").animate({ scrollTop: 0 }, "slow");
});
3. Form Submit & Reset
The following snippet will submit a form using jQuery.
$("#form_id")[0].submit();
How to reset a form:
$("#form_id")[0].reset();
Prevent from submitting the form more than once:
$("#form_id")[0].submit(function(event) {
$("#form_id")[0].submit(function(event) {
return false;
});
});
4. Input Validation
The following snippets will add validation to your input fields.
Email validation:if (/^([a-zA-Z0-9_.+-])+\@(([a-zA-Z0-9-])+\.)+([a-zA-Z0-9]{2,4})+$/.test($("#email").val())) {
console.log("Input field has valid email!");
}
Length validation:
if ($("#password").val().length < 5 || $("#password").val().length > 15) {
console.log("Password must be between 5 and 15 characters long!");
}
Username validation:
if (!/^[a-zA-Z0-9]+$/.test($("#username").val())) {
console.log("Username must contain only numbers and characters!");
}
5. Download File
The following snippets will download a file.
Download file from a URL:var element = document.createElement("a");
element.setAttribute("href", "https://codeshack.io/web/img/codeshack.png");
element.setAttribute("download", "codeshack.png");
document.body.appendChild(element);
element.click();
Download textarea text:
var element = document.createElement("a");
element.setAttribute("href", "data:text/plain;charset=utf-8," + encodeURIComponent($("#textarea").val()));
element.setAttribute("download", "textarea-text.txt");
document.body.appendChild(element);
element.click();
6. Prevent Right Click
The following snippet prevents the user from right clicking your page, which is ideal if you want to stop the user from copying content, etc.
$(document).bind("contextmenu", function(event) {
return false;
});
7. Get Widths & Heights
The following snippets will get the width and height of elements.
Browser view-port:$(window).width();
$(window).height();
HTML Document:
$(document).width();
$(document).height();
Specific element:
$("#element_id").width();
$("#element_id").height();
Screen size:
screen.width;
screen.height;
8. Check if Element Exists
The following snippet will check if an element exists. The majority of people tend to forget, so I thought I'd throw it in.
if ($("#element_id").length) {
console.log("Element exists...");
} else {
console.log("Element does not exists...")
}
9. String Manipulation
The following snippets will show you how to manipulate strings.
Replace a word:$("#element_id").val().replace("Dave", "David");
Case sensitivity:
$("#element_id").val().toLowerCase();
$("#element_id").val().toUpperCase();
String length:
$("#element_id").val().length;
Remove white-spaces from the start and end of the string:
$("#element_id").val().trim();
Convert string to an array:
$("#element_id").val().split(" ");
Check if a string contains another string:
$("#element_id").val().contains("dave");
10. Add Items to List
The following snippets will show you how you can add items to your lists, tables, etc.
Add item to list:$("ul").append("<li>List Item " + $("ul li").length + "</li>");
Add item to table:
$("table").append("<tr><td>item 1</td><td>item 2</td></tr>");
Add item to the start of a list:
$("ul").prepend("<li>List Item " + $("ul li").length + "</li>");
11. Check if Element is Visible or Hidden
The following snippet will show you how to check if an element is hidden or visible.
if ($("#element_id").is(":visible")) {
console.log("Element is visible...");
} else {
console.log("Element is hidden...");
}
12. Get the Selected Radio Button
The following snippet will show you how to get the selected radio button.
$("#form_id input[type='radio']:checked").val();
13. Check if Checkbox is Checked
The following snippet will check if a checkbox is checked.
if ($("#element_id").is(':checked'))) {
console.log("Checkbox is checked...");
} else {
console.log("Checkbox is unchecked...");
}
14. Get IP Address
The following snippet will show you how to get the IP address of the client.
$.get('http://jsonip.com', function(data) {
console.log(data.ip);
});
15. Countdown in Seconds
The following snippet will countdown in seconds.
HTML code:<p id="countdown">60</p>
jQuery code:
var countdown = setInterval(function() {
var num = parseInt($("#countdown").text());
if (num == 0) {
clearInterval(countdown);
} else {
$("#countdown").text(num - 1)
}
}, 1000);
16. Copy Text from Textarea
The following snippet will select and copy text from a textarea.
$("textarea").select();
document.execCommand('copy');
17. Mobile Device Detection
The following snippet will detect if the visitor is using a mobile device.
if(/Android|webOS|iPhone|iPad|iPod|BlackBerry|IEMobile|Opera Mini/i.test(navigator.userAgent)) {
console.log("This device is a mobile.");
}
18. Redirect Page after Specific Time
The following snippet will redirect the document page after 5 seconds.
window.setTimeout(function() {
window.location.href = "http://www.codeshack.io";
}, 5000);
19. Refresh Page
The following snippet will refresh/reload the current page.
location.reload();
20. Selecting Elements with Data Attribute
The following snippets will show you how to select elements that contain the data attributes.
Select all elements containing the attribute data-myattr:$('[data-myattr]');
Select all elements containing the attribute data-myattr along with the value set to CodeShack:
$('[data-myattr="CodeShack"]');
Conclusion
That's basically my list of goto snippets for my projects that require jQuery.
Feel free to drop a comment and let us know your favorite snippets.
If you've enjoyed reading this article, share it with social media using the social links below as it helps us create more quality content.
Enjoy coding!