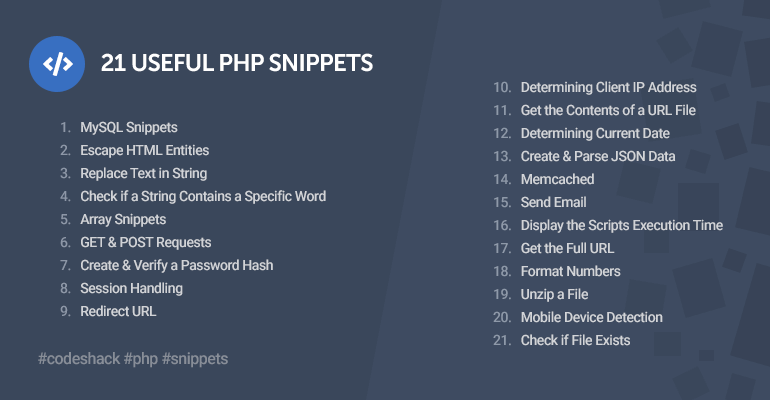
A comprehensive list of useful PHP snippets that will enable you to implement them into your projects with minimal effort.
For optimal performance and compatibility, use the latest version of PHP.
Who can use the PHP snippets?
Anyone who is planning on creating web applications with the server-side language PHP, whether you're a beginner or advanced programmer, we have a diverse set of snippets readily available for you.
Contents
- MySQL Snippets
- Escape HTML Entities
- Replace Text in String
- Check if a String Contains a Specific Word
- Array Snippets
- GET and POST Requests
- Create and Verify a Password Hash
- Session Handling
- Redirect URL
- Determining Client IP Address
- Get the Contents of a URL File
- Determining Current Date
- Create and Parse JSON Data
- Memcached
- Send Email
- Display the Scripts Execution Time
- Get the Full URL
- Format Numbers
- Unzip a File
- Mobile Device Detection
- Check if File Exists
1. MySQL Snippets
The following snippet will connect to your MySQL database using the MySQL improved extension.
$mysqli = mysqli_connect('localhost', 'DATABASE_USERNAME', 'DATABASE_PASSWORD', 'DATABASE_NAME');
However, for the above connection code to work correctly, you must update the parameters to reflect your database credentials.
Check and output connection errors:
if (mysqli_connect_errno()) {
exit('Failed to connect to MySQL: ' . mysqli_connect_error());
}
Select database table and populate the results:
$result = $mysqli->query('SELECT * FROM students');
while ($row = $result->fetch_assoc()) {
echo $row['name'] . '<br>';
}
Retrieve the number of rows:
$result->num_rows;
Insert a new record:
$mysqli->query('INSERT INTO students (name) VALUES ("David")');
Retrieve the number of affected rows:
$mysqli->affected_rows;
Escape special characters in a string (which is vital if you're not using prepared statements):
$mysqli->real_escape_string($user_input_text);
Prepare statement (prevents SQL injection):
$name = 'David';
$limit = 1;
// Prepare query
$stmt = $mysqli->prepare('SELECT age, address FROM students WHERE name = ? LIMIT ?');
// data types: i = integer, s = string, d = double, b = blog
$stmt->bind_param('si', $name, $limit);
// Execute query
$stmt->execute();
// Bind the result
$stmt->bind_result($age, address);
Close the query and database connection:
$stmt->close();
$mysqli->close();
2. Escape HTML Entities
The following snippet will escape HTML entities and quotes, which will prevent XSS attacks.
htmlentities($text, ENT_QUOTES, 'UTF-8');
Decoding HTML entities:
html_entity_decode($text);
3. Replace Text in String
The following snippet will replace text in a string.
str_replace('Apple', 'Orange', 'My favourite fruit is an Apple.');
And to replace multiple words in a string, we can declare an array of strings:
str_replace(array('fruit', 'Apple'), array('Vegetable', 'Carrot'), 'My favourite fruit is an Apple.');
4. Check if a String Contains a Specific Word
The following snippet will check if a string contains a specific word:
if (strpos('My name is David.', 'David') !== false) {
// String contains David
}
With PHP >= 8, we can do:
if (str_contains('My name is David.', 'David')) {
// String contains David
}
5. Array Snippets
Inserting new items into an array:
$names = array();
array_push($names, 'David', 'John');
We can also do:
$names = array();
$names[] = 'David';
$names[] = 'John';
Remove item from an array:
unset($names['David']);
// or...
unset($names[0]);
Reindex the values after you have removed an item:
$names = array_values($names);
Reverse an array:
$reversed = array_reverse($names);
Merge two or more arrays:
$merged_array = array_merge($array1, $array2);
Return only the array keys:
$keys = array_keys(array('name' => 'David', 'age' => 28));
Sort an array in ascending order:
sort($names);
Sort an array in reverse order:
rsort($names);
Check if an item exists in an array:
if (in_array('David', $names)) {
// item exists
}
Check if key exists in an array:
if (array_key_exists('name', array('name' => 'David', 'age' => 28))) {
// key exists
}
Count the number of items in an array:
count($names);
Convert comma-separated list to array:
$array = explode(',', 'david,john,warren,tracy');
6. GET and POST Requests
Retrieve a GET request parameter, which can be specified in the URL:
// index.php?name=david
echo $_GET['name'];
// index.php?name=david&surname=adams
echo $_GET['surname'];
Check if request variable exists:
if (isset($_GET['name'])) {
// exists
}
Process HTML form data with PHP:
<form action="login.php" method="post">
Username: <input type="text" name="username"><br>
Password: <input type="password" name="password"><br>
<input type="submit">
</form>
login.php:
$user = $_POST['username'];
$pass = $_POST['password'];
echo 'Your username is ' . $user . ' and your password is ' . $pass . '.';
7. Create and Verify a Password Hash
The following snippet will create a password hash using a one-way hashing algorithm.
$hash = password_hash('your_password', PASSWORD_DEFAULT);
Verify your hashed passwords:
if (password_verify('your_password', $hash)) {
// hash is valid
}
8. Session Handling
In a nutshell, sessions act as cookies but are stored on the server as opposed to the client's computer. In addition, a unique ID is generated and stored as a cookie in the client's browser. It's used to associate the client with the session data.
The following snippet will create a new session.
session_start();
$_SESSION['name'] = 'David';
Free all the session variables associated with the client from memory:
session_unset();
Destroy all the session variables associated with the client:
session_destroy();
9. Redirect URL
The following snippet will redirect to a new page:
header('Location: http://example.com/newpage.php');
10. Determining Client IP Address
This snippet will get the IP address of the client:
if (!empty($_SERVER['HTTP_CLIENT_IP'])) {
$ip = $_SERVER['HTTP_CLIENT_IP'];
} elseif (!empty($_SERVER['HTTP_X_FORWARDED_FOR'])) {
$ip = $_SERVER['HTTP_X_FORWARDED_FOR'];
} else {
$ip = $_SERVER['REMOTE_ADDR'];
}
echo $ip; // outputs the IP address to screen
11. Get the Contents of a URL File
The following snippet will get the contents of a file using cURL.
$url = 'http://example.com/file.json';
$curl = curl_init();
curl_setopt($curl, CURLOPT_URL, $url);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
curl_setopt($curl, CURLOPT_HEADER, false);
curl_setopt($curl, CURLOPT_FOLLOWLOCATION, true);
curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, false);
$result = curl_exec($curl);
curl_close($curl);
Using the PHP file_get_contents function:
$result = file_get_contents('file.json');
12. Determining Current Date
The following snippet will get the current date in string format.
$date = date('Y-m-d H:i:s');
echo $date;
Get the date from a timestamp:
$timestamp = 1641826797;
$date = date('Y-m-d H:i:s', $timestamp);
echo $date;
13. Create and Parse JSON
The following snippet will convert an PHP array to JSON.
$array = array('name' => 'David', 'age' => 27);
$string = json_encode($array);
echo $string; // { "name" : "David", "age" : 27 }
Parse an JSON file:
$string = file_get_contents('file.json');
$json = json_decode($string, true);
// $json['name'] etc...
14. Memcached
Memached is an extension that temporarily stores data in memory. It is useful for demanding applications.
Connect to memcached server:
$memcached = new Memcached();
$memcached->addServer('127.0.0.1', 11211);
Set item:
$memcached->set('name', 'David');
Get item:
$memcached->get('name');
15. Send Email
The following snippet will send an email.
$from = 'david@yourwebsite.com';
$to = 'nobody@example.com';
$subject = 'Your Subject';
// Plain message
$message = 'Hello! How are you today?';
// HTML message
$message = '<p>Hello! How are you today?</p>';
$headers = 'From: ' . $from . "\r\n" . 'Reply-To: ' . $from . "\r\n" . 'Return-Path: ' . $from . "\r\n" . 'X-Mailer: PHP/' . phpversion() . "\r\n" . 'MIME-Version: 1.0' . "\r\n" . 'Content-Type: text/html; charset=UTF-8' . "\r\n";
mail($to, $subject, $message, $headers);
16. Display the Scripts Execution Time
The following snippet will display the scripts execution time.
$time_start = microtime(true);
// Place your scripts here...
echo 'Total execution time in seconds: ' . (microtime(true) - $time_start);
17. Get the Full URL
The following snippet will get the full URL of your website (replace HTTP with HTTPS if using SSL).
$url = 'http://' . $_SERVER['HTTP_HOST'] . $_SERVER['REQUEST_URI'];
18. Format Numbers
The following snippet will format a number.
$num = number_format(1000);
echo $num; // 1,000
Format number with decimals:
$num = number_format(1000.12, 2);
echo $num; // 1,000.12
19. Unzip a File
The ZIP extension is required for this to work.
The following snippet will unzip an archive file on your server.
$zip = new ZipArchive;
$file = $zip->open('file.zip');
if ($file) {
$zip->extractTo('/extract_path/');
$zip->close();
echo 'Archive extracted successfully!';
}
20. Mobile Device Detection
The following snippet will detect if the client is using a mobile device.
if (preg_match("/(android|avantgo|blackberry|bolt|boost|cricket|docomo|fone|hiptop|mini|mobi|palm|phone|pie|tablet|up\.browser|up\.link|webos|wos)/i", $_SERVER["HTTP_USER_AGENT"])) {
// Is mobile...
}
21. Check if File Exists
The following snippet will check if a file exists.
if (file_exists('example.png')) {
// File exists
}
Conclusion
These snippets are useful if you develop PHP applications regularly or if you're a beginner programmer looking for a particular snippet. Keep the page bookmarked for future reference.
If you've enjoyed reading this article, consider sharing it on social media using the social links below. It will help us provide you with more quality content.
Enjoy coding!