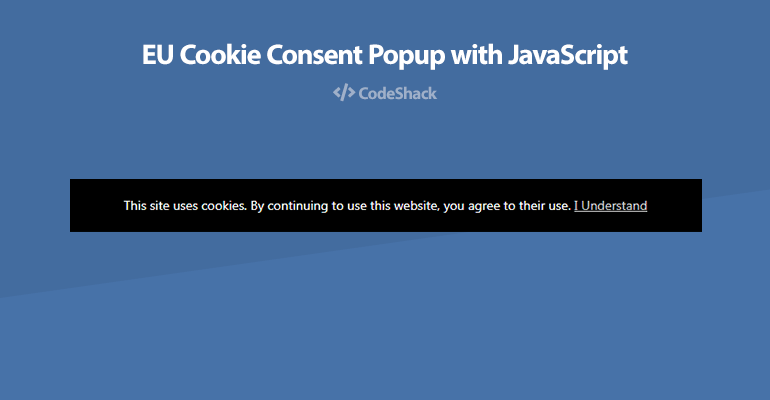
By law if you own a website and it uses cookies you need your visitors to consent to them, this currently only applies to EU visitors, visitors outside of the EU don't need to agree to use cookies on your website.
The solution? A simple popup that appears on your website, this popup will let the visitors know if they consent to the use of cookies.
How do I do this? With JavaScript — If a visitor agrees to the use of cookies, JavaScript can store the "agreement" so the visitor doesn't have to agree on every page they visit.
The script:
(function() {
if (!localStorage.getItem('cookieconsent')) {
var request = new XMLHttpRequest();
request.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
var data = JSON.parse(request.responseText);
var eu_country_codes = ['AL','AD','AM','AT','BY','BE','BA','BG','CH','CY','CZ','DE','DK','EE','ES','FO','FI','FR','GB','GE','GI','GR','HU','HR','IE','IS','IT','LT','LU','LV','MC','MK','MT','NO','NL','PO','PT','RO','RU','SE','SI','SK','SM','TR','UA','VA'];
if (eu_country_codes.indexOf(data.countryCode) != -1) {
document.body.innerHTML += '\
<div class="cookieconsent" style="position:fixed;padding:20px;left:0;bottom:0;background-color:#000;color:#FFF;text-align:center;width:100%;z-index:99999;">\
This site uses cookies. By continuing to use this website, you agree to their use. \
<a href="#" style="color:#CCCCCC;">I Understand</a>\
</div>\
';
document.querySelector('.cookieconsent a').onclick = function(e) {
e.preventDefault();
document.querySelector('.cookieconsent').style.display = 'none';
localStorage.setItem('cookieconsent', true);
};
}
}
};
request.open('GET', 'http://ip-api.com/json', true);
request.send();
}
})();
With the above solution we get the visitors geolocation info with ip-api, we can get the country code and this is what we can use to check if the visitor is in the EU, if you would like to consent more countries you could add the country code to the eu_country_codes array.
Remember to put this code at the end of your document just before the body close tag, this will ensure that it works correctly.
If we want everybody to agree to the use of cookies, we can simply do:
(function() {
if (!localStorage.getItem('cookieconsent')) {
document.body.innerHTML += '\
<div class="cookieconsent" style="position:fixed;padding:20px;left:0;bottom:0;background-color:#000;color:#FFF;text-align:center;width:100%;z-index:99999;">\
This site uses cookies. By continuing to use this website, you agree to their use. \
<a href="#" style="color:#CCCCCC;">I Understand</a>\
</div>\
';
document.querySelector('.cookieconsent a').onclick = function(e) {
e.preventDefault();
document.querySelector('.cookieconsent').style.display = 'none';
localStorage.setItem('cookieconsent', true);
};
}
})();
The upside to this is that it doesn't need to rely on an external API.
The output:
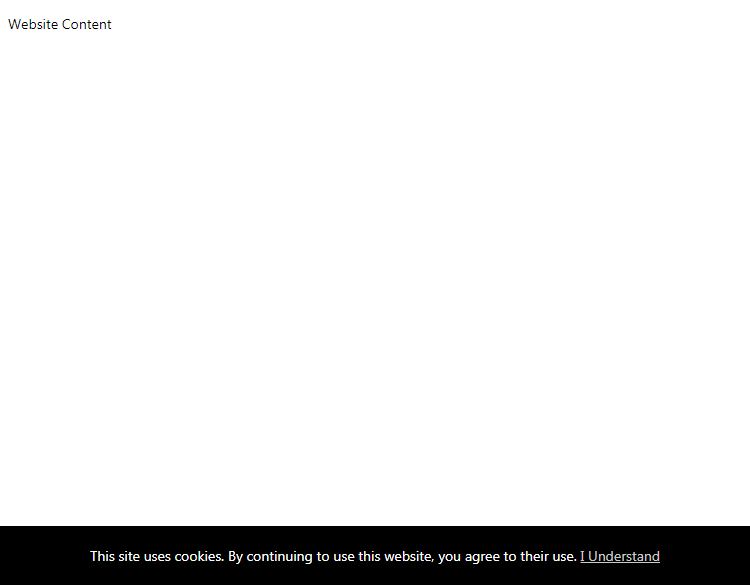
Conclusion
The whole ordeal with the cookie consent can be a tad annoying, the solution above will implement the cookie consent for you and save you the time, you don't need any external cookie consent scripts, no external libraries (jQuery, etc), this is pure JavaScript.
You're totally free to use the scripts above, no attribution required, enjoy!