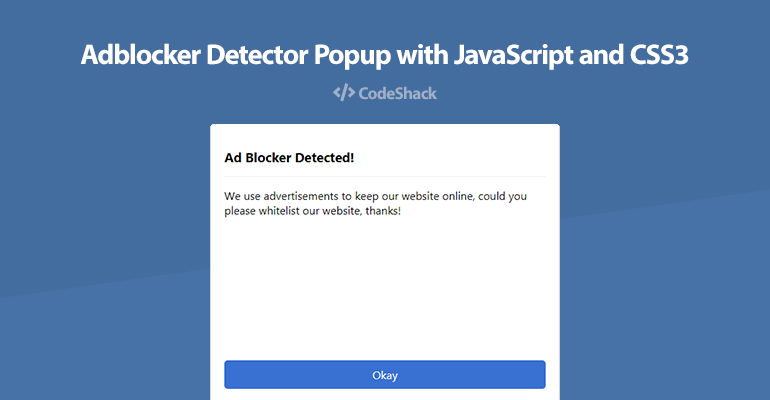
Did you know roughly 30% of internet users use an adblocker? If you use advertisements on your website to cover the costs of running it you could potentially be losing revenue.
The solution? You cannot stop your visitors from using an adblocker but you can politely ask them to whitelist your website.
Place the below JS code in your JS file or on the page you would like to show the popup.
fetch('http://pagead2.googlesyndication.com/pagead/js/adsbygoogle.js').catch(() => {
let adp_underlay = document.createElement('div');
adp_underlay.className = 'adp-underlay';
document.body.appendChild(adp_underlay);
let adp = document.createElement('div');
adp.className = 'adp';
adp.innerHTML = `
<h3>Ad Blocker Detected!</h3>
<p>We use advertisements to keep our website online, could you please whitelist our website, thanks!</p>
<a href="#">Okay</a>
`;
document.body.appendChild(adp);
adp.querySelector('a').onclick = e => {
e.preventDefault();
document.body.removeChild(adp_underlay);
document.body.removeChild(adp);
};
});
Using the fetch method we can fetch the Google AdSense script, most adblockers will block this script as it is the most common resource for advertisements, the catch callback statement will check if the HTTP response has failed, basically meaning the resource has been blocked by an adblocker.
If the response has failed to load the code will add the popup box to the document, feel free to change the message and title.
The CSS code (add to your stylesheet):
.adp {
display: flex;
box-sizing: border-box;
flex-flow: column;
position: fixed;
z-index: 99999;
left: 50%;
top: 50%;
transform: translate(-50%, -50%);
width: 500px;
height: 400px;
background-color: #ffffff;
padding: 20px;
border-radius: 5px;
}
.adp h3 {
border-bottom: 1px solid #eee;
margin: 0;
padding: 15px 0;
}
.adp p {
flex-grow: 1;
}
.adp a {
display: block;
text-decoration: none;
width: 100%;
background-color: #366ed8;
text-align: center;
padding: 10px;
box-sizing: border-box;
color: #ffffff;
border-radius: 5px;
}
.adp a:hover {
background-color: #3368cc;
}
.adp-underlay {
background-color: rgba(0, 0, 0, 0.5);
position: fixed;
width: 100%;
height: 100%;
top: 0;
left: 0;
z-index: 99998;
}
The result will look like the following if the user has their Adblock enabled:
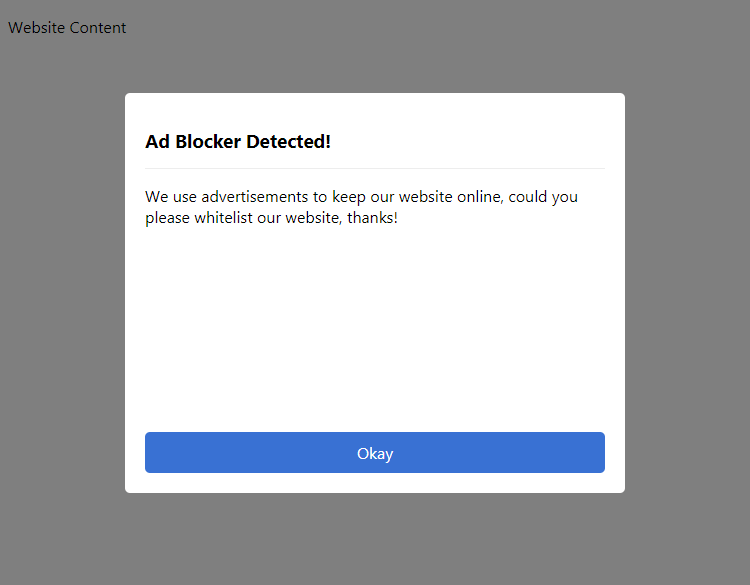
Conclusion
That's how you create a basic popup for Adblock users, feel free to use the code on your website and/or projects, just remember not to rename the class name to anything like "adbanner" or "adblock" because some ad blockers are able to detect this and hide them from the user.
If you enjoyed this article don't forget to share with your friends and drop a comment below. If you're interested in programming, check out our other articles.